Difference between Clone and Copy
Clone and Copy ?
Clone will copy the structure of a data where as Copy will copy the complete structure as well as data.
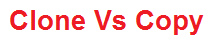
Consider this string[] example:
Here declare two string array arr1 and arr2 and then copy arr1 to arr2.
The arr2 now contains the same values, but more importantly the array object itself points to the same object reference as the arr1 array.
Here now assign the value to arr2
Now display the values in message box, What would be the output for the following statements?
Considering both arrays point to the same reference we would get:
Form the next sample code you can understand how clone() is working.
The arr4 now contains the same values, but in this case the array object itself points a different reference than the arr3.
What would be the output now for the following statements?
Differences between dataset.clone and dataset.copy ?
Both the dataset.Copy and the dataset.Clone methods create a new DataTable with the same structure as the original DataTable. The new DataTable created by the Copy method has the same set of DataRows as the original table, but the new DataTable created by the Clone method does not contain any DataRows
It will copy all the data and structure to dt table.
It will create only the structure of table not data.
- Difference between NameSpace and Assembly
- Execution process for managed code
- What is an Asssembly Qualified Name
- How to Assembly versioning
- Add and remove an assembly from GAC
- What is Native Image Generator (Ngen.exe)?
- How to force Garbage Collection
- Difference between CType and DirectCast
- Obsolete or Deprecated in NET Framework
- What is lazy initialization
- Difference between Finalize() and Dispose()
- Difference between static and constant